Ensuring the accuracy and validity of user input is paramount, especially in web forms where address validation plays a critical role. Utilizing regular expressions (regex) in JavaScript is an effective method for achieving this.
In this guide about address validation regex javascript, we’ll cover regex basics, how to craft patterns for various address components, and explore advanced features for robust address validation.
By the end of this article, you will be fully prepared to implement regex-based address validation in your web applications, significantly enhancing user experience and data integrity.
💡 GeoPostcodes offers comprehensive and accurate postal and zip code data to ensure the reliability of address validation, which is more accurate and reliable than regex. Browse GeoPostcodes datasets and download a free sample here.
Prior to exploring the guide, it’s important to mention that using regex to validate an address is generally not advisable due to the diverse address formats worldwide.
Assumptions
This subject is complex, and a perfect solution using only regular expressions is impossible. To validate an address with perfect accuracy, you must use an accurate and updated location database like GeoPostcodes.
To achieve a good result, this article makes several assumptions:
- only US addresses are validated
- the address is written without too many errors
- the address is in the format “{number} {street} {town}, {state} {zip}, United States”
The Basics of Regex for Address Validation
Testing using the right tool
Whether you are an expert or a beginner at writing regular expression, I recommend using this website:
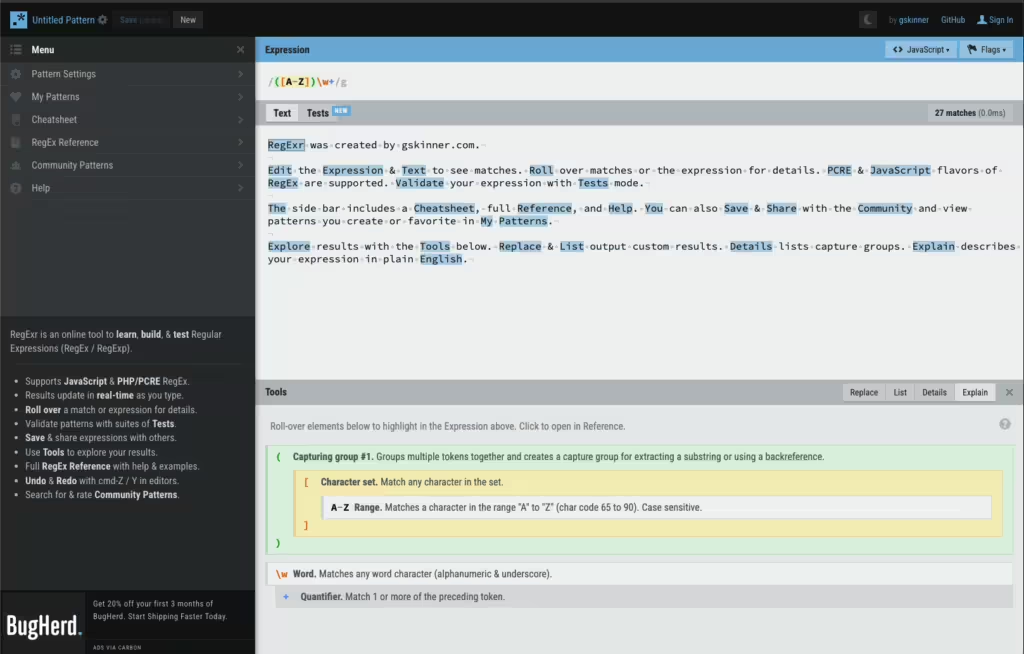
It’s a powerful and user-friendly tool for learning, testing, and debugging regular expressions. It offers an intuitive interface that allows you to experiment with regex patterns in real time, highlighting matches and providing detailed explanations of each part of the pattern. RegExr makes complex pattern matching more approachable, making it a must-use for anyone working with regular expressions in programming or data manipulation.
Understanding Regex Syntax
To effectively use regular expressions for address validation, it’s essential to understand the basic syntax and components of a regex pattern. A regex pattern is a sequence of characters that defines a search pattern. Here are some key elements to grasp:
- Anchors: These are used to specify the position of the match within the string. For example, ^ denotes the start of the string, and $ denotes the end of the string. In the context of email validation, the pattern ^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$ uses these anchors to ensure the entire string matches the pattern. This is achieved by starting the expression with the “start of the string” anchor and ending with the “end of the string” anchor.
- Character Classes: These define sets of characters that can match a single character in the string. For instance, [a-zA-Z0-9_.+-] matches any letter (uppercase and lowercase), digit, underscore, dot, plus sign, or hyphen. Some character classes have a shortcut. For example, the number ([0-9]) can be shortened to \d and white space characters to \s.
- Quantifiers: They specify the number of times a preceding element should be matched. Common quantifiers include ? (zero or one), + (one or more), * (zero or more), and {n, m} (between n and m times; note that the “n” part is optional). It’s possible to match as few characters as possible with the quantifier +?.
- Special Characters: Some characters have special meanings in regex, such as . (matches any character except a newline), \ (escape character), and | (alternation). These characters need to be escaped if they are to be matched literally.
- Capturing group: A pattern can be put in parentheses to be grouped. This allows the matching sequence to be referenced.
Constructing Regex for Different Address Components
To ensure accuracy, this article will adhere to the USPS guidelines for formatting US addresses, structured as follows: “{number} {street} {town}, {state} {zip}, United States.”
Let’s create the regular expression element by element.
Number
^\\d+[A-Z]*
This will match a sequence of numbers at the start of the text with optional letters at the end to account for numbers ending with an “a.” It is important to remember that it does not always start with a number and the slash (/) is also allowed.
Street
[A-Z0-9\\s.-]+?
This will match a sequence with as few characters as possible composed of:
- a letter or
- a number or
- a space or
- a dot or
- a dash
This will match a wide variety of text on purpose, as the street can use many different characters. Please note that, for street names, the slash (/) and the apostrophe (‘) are also permitted.
Town and state
[A-Z\\s.-]+?
The town and the state can use the same detection pattern, as they are similar in terms of possible values. Please note that an apostrophe followed by a comma is only permitted for “Washington, D.C.” Also, Doña Ana County requires a special case.
Compared to the street expression, it’s more restrictive. It only checks for characters, spaces, dots, or dashes. Again, this will match as few characters as possible.
Zip
\\d{5}
The zip code is the easiest to validate (except when ZIP+4 is used) as it’s always a sequence of 5 numbers.
Everything put together
Now that we have established what each part should be let’s combine them into one expression by concatenating them.
^(\\d+[A-Z]*)\\s([A-Z0-9\\s.-]+?)[\\s,]+([A-Z\\s.-]+?)[\\s,]+([A-Z\\s.-]+)\\s(\\d{5})[\\s,]+(United States(?: Of America)?)$
There are several things to notice.
- This expression assumes that you are executing the regular expression as case-insensitive (see the paragraph below on flag modifiers).
- To be as wide as possible, the separation between elements is defined as: [\s,]+. This will match one or multiple spaces or commas. So, for example, all these strings will match:
,
,,
,,
, or . - The address should end with United States, but it could also be United States of America. The expression at the end accounts for both possibilities: United States( Of America)?.
- This uses capturing groups so that a valid address can be decomposed into its parts for later processing. Note: This will not work without a comma separating the elements of the address, making it difficult to distinguish between the street and the town.
Examples
Let’s use valid examples to showcase the full regular expression and the capturing groups that come out of it:
1600 Pennsylvania Avenue NW, Washington, D.C. 20500, United States
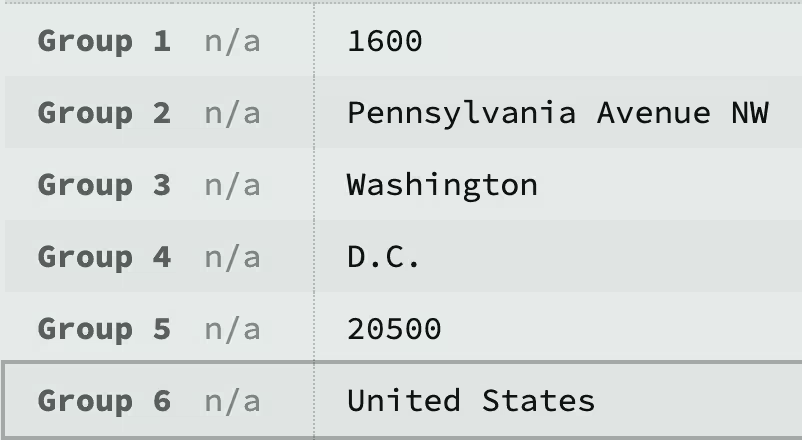
Here is the list of what each group corresponds to:
- Group 1: number
- Group 2: street
- Group 3: town
- Group 4: state
- Group 5: zip
- Group 6: country
Another example of a valid address being parsed:
20 W 34th St., New York, NY 10001, United States
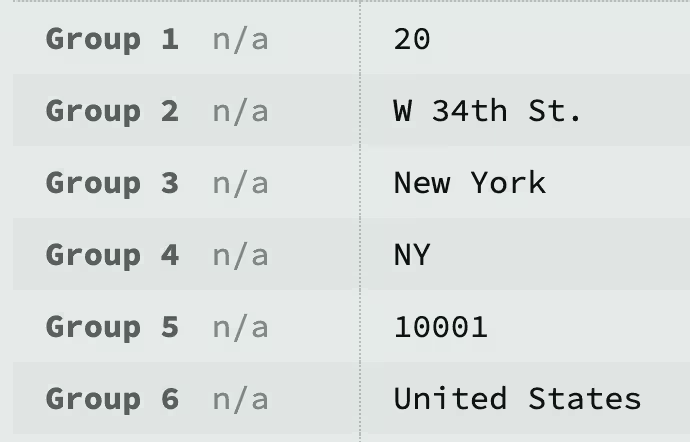
Advanced Javascript Regex Features for Robust Address Validation
Using Flags and Modifiers
When working with regular expressions for address validation, utilizing flags and modifiers can significantly enhance the flexibility and accuracy of your patterns. Here are some key flags and modifiers you should be aware of:
- Case Insensitivity: The i flag makes the regex pattern case-insensitive, which is essential for email and other address validations where the case does not matter. For example, you can use RegExp(‘pattern’, ‘i’) in JavaScript to make the pattern case-insensitive.
- Multiline Mode: The m flag changes the behavior of the ^ and $ anchors to match the start and end of each line rather than the entire string. This can be useful when dealing with multiple addresses.
- Global Match: The g flag in JavaScript allows the regex to find all matches in a string rather than stopping after the first match. This is particularly useful when validating multiple addresses in a single input field.
- Sticky Flag: The y flag in JavaScript ensures that the regex matches only from the index indicated by the lastIndex property of the regex object. This can help validate addresses sequentially.
Optimizing Regex Performance
While regular expressions are powerful tools for pattern matching, poorly constructed or overly complex patterns can lead to performance issues. Here are some tips to optimize the performance of your regex patterns:
- Avoid Excessive Use of * and +: These quantifiers can lead to catastrophic backtracking, significantly slowing down the regex engine. Instead, use more specific quantifiers like {n, m} where possible.
- Use Anchors Wisely: Anchors like ^ and $ can help the regex engine quickly determine if a match is possible, reducing unnecessary processing. Ensure that your patterns are anchored appropriately to the start or end of the string.
- Minimize Backtracking: Complex patterns with many alternatives can cause the regex engine to backtrack excessively, leading to performance issues. Simplify your patterns and use non-capturing groups (?:) instead of capturing groups () when unnecessary.
- Test and Refine: Always test your regex patterns with various inputs to ensure they perform as expected. Based on the results, refine your patterns to optimize performance and accuracy.
Conclusion
In conclusion, mastering the art of address validation using regex in JavaScript is a powerful tool for ensuring data accuracy and enhancing user experience.
It’s essential to understand the basics of regex syntax, construct patterns for different address components, and utilize flags and modifiers to optimize performance.
GeoPostcodes offers comprehensive and accurate postal and zip code data to ensure the reliability of address validation. Browse GeoPostcodes datasets and download a free sample here.
FAQ
How to validate the address field in JavaScript?
To validate the address field in JavaScript, it’s essential to understand the basics of regex syntax.
Construct patterns for different address components and utilize flags and modifiers to optimize performance.
How to make regex validation in JavaScript?
Understanding the basic syntax and components of a regex pattern is essential for effectively using regular expressions.
How to use regex to validate email in JavaScript?
Use the pattern: ^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$
What is the meaning of a zA z?
[a-zA-Z] matches any character from lowercase a through uppercase Z.