Introduction
Geographic coordinates to zip code conversion is a key challenge in location intelligence and address management. Businesses must regularly determine the correct zip code for specific latitude and longitude coordinates. This reverse geocoding process bridges raw geographic data with standardized address information critical for operations.
Reverse geocoding transforms precise location coordinates into human-readable address components. Zip codes hold particular value for business operations across industries. Organizations utilize this capability to power location-based services, streamline customer communications, and enhance delivery logistics. When customers access services, their device’s GPS coordinates can be converted instantly to a zip code, enabling location-specific messaging without manual address entry. The accurate ability to get a zip code from latitude and longitude drives operational efficiency.
This article explores practical methods for converting coordinates to zip codes, highlighting how GeoPostcodes’ location data solutions support better decision-making, streamlined operations, and enhanced customer experiences.
💡 For over 15 years, we have created the most comprehensive worldwide zip code database. Our location data is updated weekly, relying on more than 1,500 sources. Browse GeoPostcodes datasets and download a free sample here.
Understanding Latitude and Longitude
Geographic coordinates form the foundation of location-based services, precisely identifying any point on Earth’s surface. Understanding their components is essential to effectively converting coordinates to zip codes.
Latitude represents the angular distance between a point on Earth’s surface and the equator, measured in degrees. Points north of the equator have positive latitude values (0° to 90°), while locations south have negative values (0° to -90°). This measurement divides Earth into horizontal bands, with the equator as the zero-reference line.
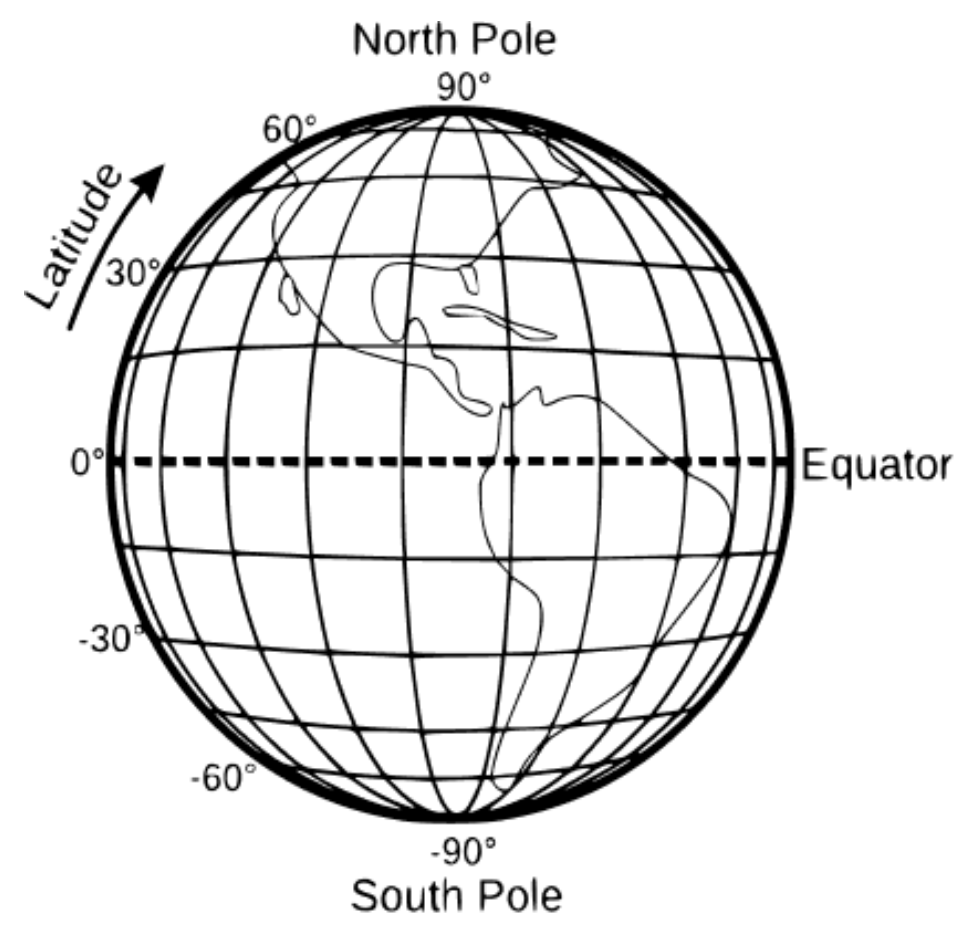
Longitude measures the angular distance east or west from the prime meridian, which runs through Greenwich, London. Longitude values range from 0° to 180° east and 0° to -180° west. Together, these measurements create a grid system enabling precise location identification. The coordinates (40.7128, -74.0060) uniquely identify New York City’s approximate center.
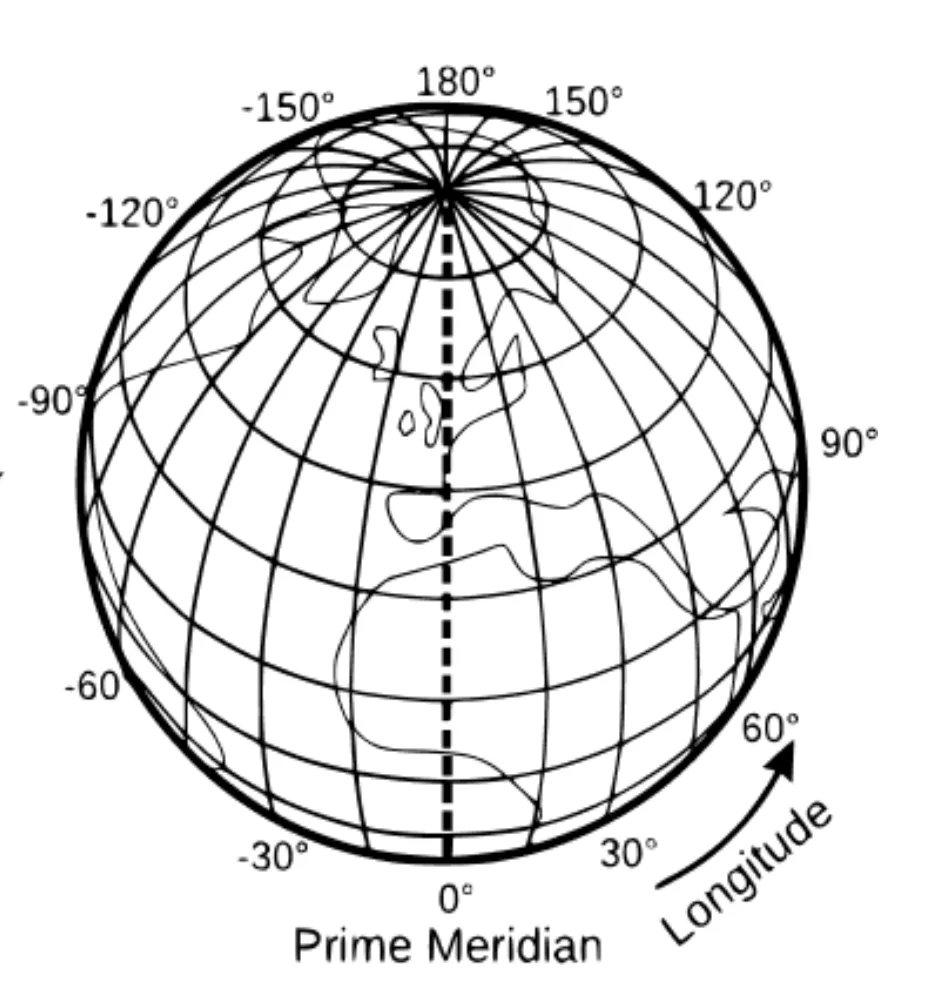
This coordinate system becomes particularly relevant when working with zip code conversion. Each zip code encompasses a specific geographic area, and determining which zip code contains a given coordinate pair requires spatial calculations based on accurate input coordinates.
Methods to Convert Latitude and Longitude to Zip Codes
Converting geographic coordinates to zip codes requires specialized tools and approaches to handle spatial data effectively. The process involves comparing the input coordinates against defined zip code boundaries or reference points to determine the appropriate zip code for a given location.
One powerful approach involves using PostgreSQL with the PostGIS extension, which provides robust spatial analysis capabilities. This combination allows for efficient geographic data processing and can handle complex spatial queries. PostGIS enhances PostgreSQL by adding support for geographic objects, enabling it to perform location queries in an SQL environment. The system can perform spatial tests to determine which zip code boundary contains a specific coordinate pair.
Consider this example of a PostGIS query that finds the zip code for a given lat and long:
SELECT zip_boundaries.postcode, point_coordinates.latitude, point_coordinates.longitude FROM zip_boundaries JOIN point_coordinates ON ST_Contains( ST_Transform(zip_boundaries.geom, 4326), ST_SetSRID(ST_MakePoint(point_coordinates.latitude, point_coordinates.longitude), 4326) );
In this query, we work with two key data elements: the zip_boundaries table storing polygon shapes of zip code areas and the point_coordinates table holding latitude/longitude data points. The ST_MakePoint function converts the coordinates to point geometry type, and the SetSRID function sets the coordinate reference system (CRS) to 4326. The polygons of all the zip codes are transformed into the same CRS. Polygons and points must have the same CRS to identify the relationship between them and the ST_Contains function accurately. That function checks if the geometry in the first argument fully contains the geometry in the second argument and returns a True or False value accordingly.
This approach uses spatial indexing for speed and efficiency, making it perfect for processing large datasets or powering real-time location lookups in customer-facing applications.
Using Python
Python offers robust capabilities for reverse geocoding operations, particularly when handling zip code boundaries at scale. Python’s geospatial libraries provide efficient tools for spatial operations, making it excellent for processing coordinate data.
We primarily rely on two powerful Python libraries for reverse geocoding with zip codes: Geopandas for handling spatial data and Shapely for geometric operations.
Geopandas extends the popular data analysis library Pandas to work with geometric types.
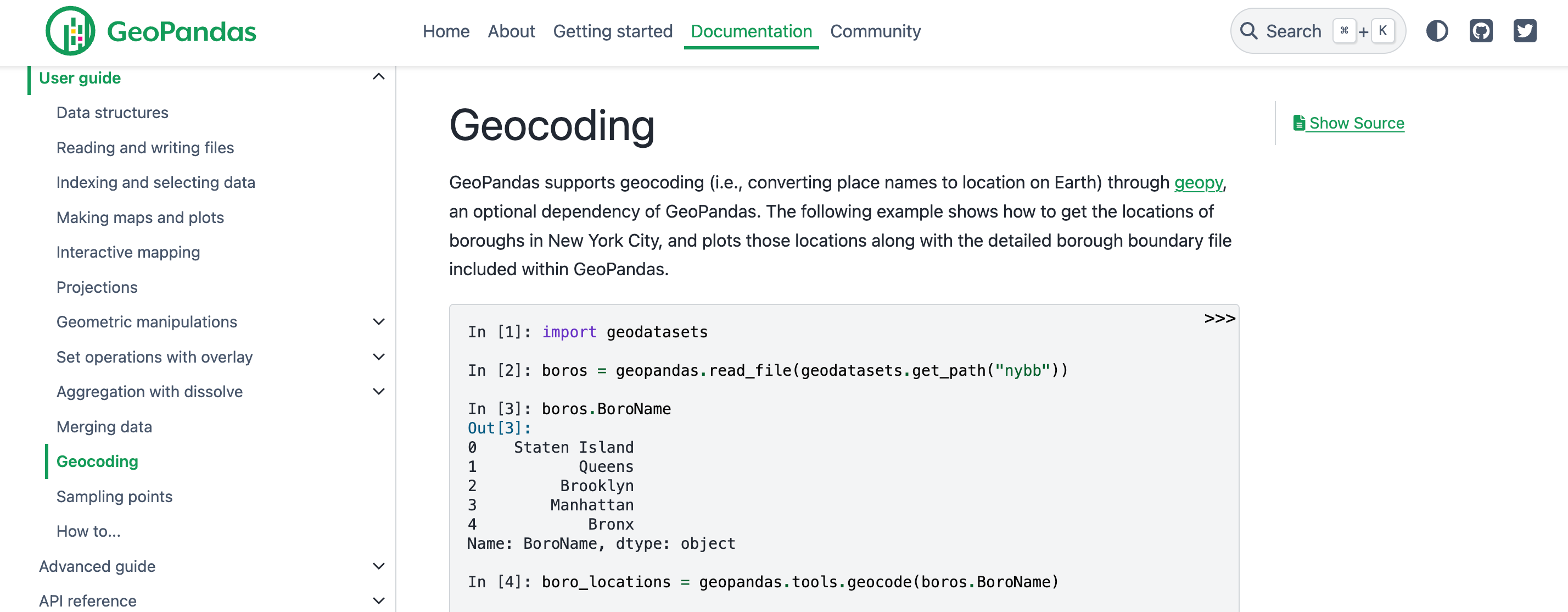
Shapely provides the fundamental geometric operations needed for spatial analysis.
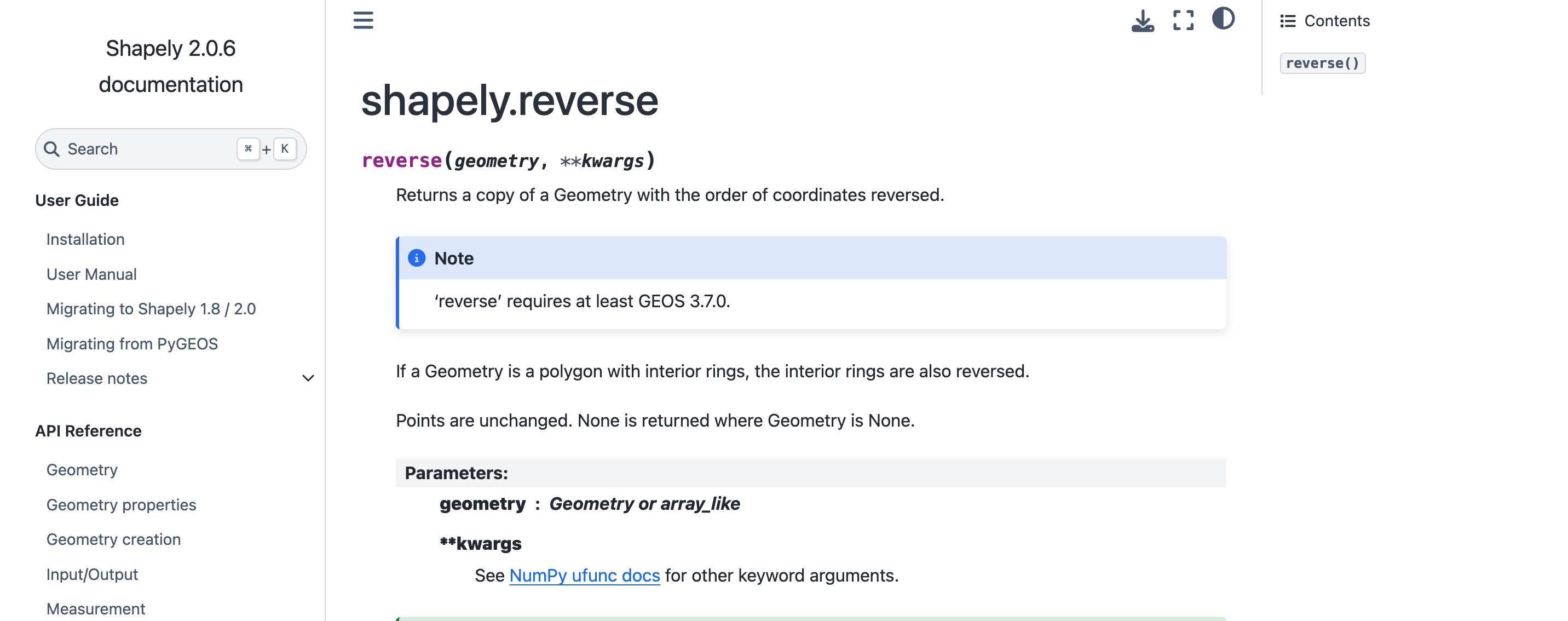
These libraries work seamlessly with standard spatial data formats, including SHP, KML, and GPKG.
You’ll need two key components to implement reverse geocoding: a dataset of coordinates you want to analyze and a comprehensive set of zip code polygons. The zip code polygons define the geographical boundaries of each zip code area. You can access free zip code polygon samples from the GeoPostcodes portal, which provides data in multiple formats to suit your needs.
import geopandas as gpd from shapely.geometry import Point import pandas as pd def load_postal_polygons(filepath): """ Load postal code polygons from a spatial file (SHP, KML, or GPKG) Args: filepath: Path to the spatial data file containing postal code polygons Returns: GeoDataFrame containing postal code polygons """ return gpd.read_file(filepath) def get_postal_code(coordinates, postal_polygons): """ Find the postal code for given coordinates Args: coordinates: tuple of (longitude, latitude) postal_polygons: GeoDataFrame containing postal code polygons Returns: postal code string or None if no match found """ # Create a Point object from the coordinates point = Point(coordinates) # Find which polygon contains the point containing_polygons = postal_polygons[postal_polygons.geometry.contains(point)] if len(containing_polygons) > 0: # Return the postal code of the containing polygon return containing_polygons.iloc[0]['postal_code'] return None def batch_reverse_geocode(coordinates_df, postal_polygons): """ Process multiple coordinates to find their postal codes Args: coordinates_df: DataFrame with 'longitude' and 'latitude' columns postal_polygons: GeoDataFrame containing postal code polygons Returns: DataFrame with original coordinates and corresponding postal codes """ # Create geometry column from coordinates geometry = [Point(xy) for xy in zip(coordinates_df['longitude'], coordinates_df['latitude'])] # Convert to GeoDataFrame points_gdf = gpd.GeoDataFrame(coordinates_df, geometry=geometry) # Perform spatial join result = gpd.sjoin(points_gdf, postal_polygons, how='left', op='within') return result # Example usage if __name__ == "__main__": # Load postal code polygons postal_polygons = load_postal_polygons('postal_codes.shp') # Single coordinate lookup coordinates = (-73.935242, 40.730610) # New York coordinates postal_code = get_postal_code(coordinates, postal_polygons) # Batch processing example coordinates_data = { 'longitude': [-73.935242, -122.419416], 'latitude': [40.730610, 37.774929] } coords_df = pd.DataFrame(coordinates_data) results = batch_reverse_geocode(coords_df, postal_polygons)
This Python implementation offers a practical approach to reverse geocoding. The solution centers on three core functions: load_postal_polygons handles data preparation, get_postal_code manages individual lookups, and batch_reverse_geocode processes multiple coordinates through efficient spatial joins.
Proper implementation requires robust coordinate validation to handle edge cases correctly. The solution supports individual lookups and batch processing, making it easily scalable and adaptable to various operational needs.
💡 Ready to convert coordinates to ZIP codes yourself? Visit the GeoPostcodes portal, navigate to Data Explorer, find Zip code & address data, and download a free sample for any country of interest. You will have a comprehensive file containing ZIP codes with their corresponding latitude and longitude coordinates—perfect for testing your implementation or enriching your location data.
Ensuring Data Accuracy
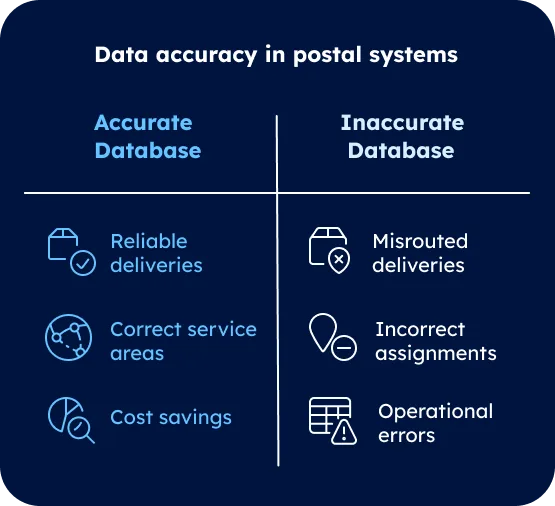
Data accuracy forms the cornerstone of reliable coordinate-to-zip code conversion systems. The quality of your results directly correlates with the precision and freshness of your reference data. Working with outdated zip code information leads to misrouted deliveries, incorrect service area assignments, and costly operational errors.
To ensure optimal accuracy in your implementations, consider creating a data validation framework that includes:
Check Type | Description | Implementation Method |
---|---|---|
Coordinate Range | Verify latitude/longitude bounds | Mathematical validation |
Data Freshness | Confirm data update frequency | Timestamp comparison |
Boundary Verification | Check point-in-polygon accuracy | Spatial analysis |
Response Validation | Verify returned zip codes | Reference comparison |
Example of coordinate range validation in Python:
def validate_coordinates(latitude, longitude): """ Validates geographic coordinates """ if not (-90 <= latitude <= 90): raise ValueError("Invalid latitude value") if not (-180 <= longitude <= 180): raise ValueError("Invalid longitude value") return True
Conclusion
Converting latitude and longitude to zip codes requires precise implementation and up-to-date reference data. We’ve explored geographic coordinate fundamentals and practical conversion techniques, highlighting their impact on business operations across industries.
Location data management demands specialized expertise and significant resources. Most organizations struggle to maintain accurate zip code databases and efficient internal conversion algorithms. Rather than building these systems from scratch, businesses benefit from accessing pre-validated, regularly updated data sources.
GeoPostcodes maintains one of the world’s most extensive location databases, covering 247 countries with weekly updates from over 1,500 authoritative sources. Our commitment to data accuracy ensures reliable coordinate-to-zip code conversions. Browse GeoPostcodes’ databases and download a free sample here.
FAQ
How do I get the address from latitude and longitude?
Use reverse geocoding to get an address from latitude and longitude coordinates. Ensure you are using a high-quality database, such as GeoPostcodes, to achieve optimal results.
How to convert latitude and longitude to address?
Use GeoPostcodes database to convert any address, street, zip code, city, administrative area, or UNLOCODE into latitude and longitude coordinates.
Why was my answer discarded when asking about ZIP codes from latitude and longitude?
ZIP codes cover areas while coordinates are points, creating ambiguity. The system may lack updated boundary data, encounter formatting issues, or face technical limitations in specific regions where postal codes aren’t clearly defined.
Why does “get zipcode from latitude and longitude” give “not the answer” I expected?
The result may vary due to data differences. Check if the coordinates are precise and match an updated database. If needed, verify results in an Excel file or cross-check with other answers in questions tagged similarly.